C# - Lesson 5b - Visual C# overview + our first real programme
+3
UF Vishna
ComitteD
UF Beazt
7 posters
Page 1 of 1
C# - Lesson 5b - Visual C# overview + our first real programme
Ok, this lesson continues on lesson 5a. There we got our project set and we dragged a button onto our form.
This is what you should have now:

Now we are going to make the form and the button look a bit better before we start the programming.
When you look on the right side at the bottom of the UI you see a 'properties' panel. Now when you select the forum itself, you will see that in that properties panel by the '(Name)' property 'Form1' is written. This is the Name you give to this form object. This means that when you are programming this "Form1" string is meant for the form object.
Note:
You can actually make it any name you want, but watch out... To prevent from misunderstandings, never write spaces in the name, and neither use underscores _ or points . ,neither any other symbol because those symbols have other programming functions and we don't want the environment to mix them up. And again, it's tradition to write you names and strings like this: myName or myStringIsThis, so always a small letter for the first word and the others you can make capitals.
Ok, you can actually leave the form's name, you don't need to change that for now. Now, when you scroll down in the form's properties list, you can see the "Text" property. That property has nothing to do with any programming. This Text is the text that will show up on the upper bar of your form. So, click it and you can change it to "Hello World" for this tutorial. You will see your form upper bar changes to "Hello World".
Now to let our form look a bit nicer we gonna change its size. Go to the size property, and change it to 250 x 100. (First make sure your button is still visible in the field after you resized the form.)
Now, we gonna change the button's size so that it fits the form. You will need to make it look huge but that doesn't matter.
You now should have something like this:
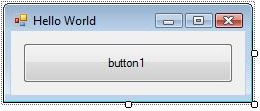
As you can see when you drag the button around, the button snaps to the corners and the edges of the form to make your UI look a bit nicer.
Now we go to the button's properties and change the Text property to 'Click Here'. So where stood 'button1' before, should now stand 'Click Here'.
Our UI is done now.
Let's start programming.
When you double click your button, the real programming code page shows up.
Your code should look like this if you haven't typed anything yet:
Your cursor will be automaticly set to the void of the object you double clicked. In this case the button.
This void will process when the button1 is clicked. (remember the name is still button1, you only changed it's Text property)
So, we want a MessageBox to show up with the words "Hello World" when we push the button.
For that we first need to call the MessageBox function.
Below is the private void of the button shown with the MessageBox function typed inside it:
Note:
In the coding window will be drawn a red line under the "MessageBox" because it has nothing to show yet. There will always show up a red line if the code is not debuggable. That means that's it's not gonna work.
You will also see the box under your word when you are typing "MessageBox", this box is the auto helper for finding your functions. This very handy to program in a fast way.
So, to continue we need to type a point behind the MessageBox function, that means "in the function MessageBox, we are looking for the subfunction ...". When you are typing the point you will see that box under it again and it gives you 3 subfunctions. (Equals, ReferenceEquals and Show) For now we are going to take the Show subfunction. That means the MessageBox will Show up. (when the button is clicked)
Now you should have this:
Now we have set enough functions. The actually content of the MessageBox comes now.
Behind the Show subfunction you gonna type this: ("Hello World")
This means the MessageBox will show you those words.
Note:
Don't forget the ; behind your MessageBox line of code. This is almost always needed in C#!
Your code should look like this now:
Now the code for your programme is set.
It's time to test it.
In the toolbar, somewhere under the button 'Data' you see this little green arrow (
)
Push that, and it will debug your code and will show your programme.
This is what is should look like when the button is pushed:

In the image the code and programme is shown.
I hope you brought this to work smoothly, and enjoyed your first programme.
You now have achieved the n00b-programmer achievement. Good job.

This is what you should have now:

Now we are going to make the form and the button look a bit better before we start the programming.
When you look on the right side at the bottom of the UI you see a 'properties' panel. Now when you select the forum itself, you will see that in that properties panel by the '(Name)' property 'Form1' is written. This is the Name you give to this form object. This means that when you are programming this "Form1" string is meant for the form object.
Note:
You can actually make it any name you want, but watch out... To prevent from misunderstandings, never write spaces in the name, and neither use underscores _ or points . ,neither any other symbol because those symbols have other programming functions and we don't want the environment to mix them up. And again, it's tradition to write you names and strings like this: myName or myStringIsThis, so always a small letter for the first word and the others you can make capitals.
Ok, you can actually leave the form's name, you don't need to change that for now. Now, when you scroll down in the form's properties list, you can see the "Text" property. That property has nothing to do with any programming. This Text is the text that will show up on the upper bar of your form. So, click it and you can change it to "Hello World" for this tutorial. You will see your form upper bar changes to "Hello World".
Now to let our form look a bit nicer we gonna change its size. Go to the size property, and change it to 250 x 100. (First make sure your button is still visible in the field after you resized the form.)
Now, we gonna change the button's size so that it fits the form. You will need to make it look huge but that doesn't matter.
You now should have something like this:
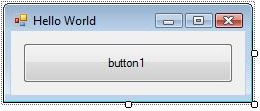
As you can see when you drag the button around, the button snaps to the corners and the edges of the form to make your UI look a bit nicer.
Now we go to the button's properties and change the Text property to 'Click Here'. So where stood 'button1' before, should now stand 'Click Here'.
Our UI is done now.
Let's start programming.
When you double click your button, the real programming code page shows up.
Your code should look like this if you haven't typed anything yet:
- Code:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace HelloWorld
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
}
}
}
Your cursor will be automaticly set to the void of the object you double clicked. In this case the button.
This void will process when the button1 is clicked. (remember the name is still button1, you only changed it's Text property)
So, we want a MessageBox to show up with the words "Hello World" when we push the button.
For that we first need to call the MessageBox function.
Below is the private void of the button shown with the MessageBox function typed inside it:
- Code:
private void button1_Click(object sender, EventArgs e)
{
MessageBox
}
Note:
In the coding window will be drawn a red line under the "MessageBox" because it has nothing to show yet. There will always show up a red line if the code is not debuggable. That means that's it's not gonna work.
You will also see the box under your word when you are typing "MessageBox", this box is the auto helper for finding your functions. This very handy to program in a fast way.
So, to continue we need to type a point behind the MessageBox function, that means "in the function MessageBox, we are looking for the subfunction ...". When you are typing the point you will see that box under it again and it gives you 3 subfunctions. (Equals, ReferenceEquals and Show) For now we are going to take the Show subfunction. That means the MessageBox will Show up. (when the button is clicked)
Now you should have this:
- Code:
private void button1_Click(object sender, EventArgs e)
{
MessageBox.Show
}
Now we have set enough functions. The actually content of the MessageBox comes now.
Behind the Show subfunction you gonna type this: ("Hello World")
This means the MessageBox will show you those words.
Note:
Don't forget the ; behind your MessageBox line of code. This is almost always needed in C#!
Your code should look like this now:
- Code:
private void button1_Click(object sender, EventArgs e)
{
MessageBox.Show("Hello World");
}
Now the code for your programme is set.
It's time to test it.
In the toolbar, somewhere under the button 'Data' you see this little green arrow (

Push that, and it will debug your code and will show your programme.
This is what is should look like when the button is pushed:

In the image the code and programme is shown.
I hope you brought this to work smoothly, and enjoyed your first programme.
You now have achieved the n00b-programmer achievement. Good job.


Guest- Guest
Re: C# - Lesson 5b - Visual C# overview + our first real programme
okey, this teached me to make my first programme, but how do I save to to .exe file type?
edit: nvm I found were it got compiled
edit: nvm I found were it got compiled
UF Beazt- UF Member
- Posts : 968
Join date : 2009-09-11
Age : 29
Location : Helsinki
Re: C# - Lesson 5b - Visual C# overview + our first real programme
in the bin map of your project ^^
Guest- Guest
coo;
cool, I am happy to see that this clan is doing stuff like that. Thats the reason I joined i4Ni before this to be with my designer friends. I wished I would have joined UF instead of i4Ni before. At least I am here now:)
ComitteD- Guest
- Posts : 19
Join date : 2011-06-02
Re: C# - Lesson 5b - Visual C# overview + our first real programme
And your welcome to stay here. . .
Btw are you doing web design?

Btw are you doing web design?
UF Vishna- UF Member
- Posts : 560
Join date : 2010-04-30
Age : 30
Location : Estonia
Re: C# - Lesson 5b - Visual C# overview + our first real programme
Not sure is anyone doing really this stuff. Xorax wrote this tutorial several months ago. I tried to learn at start but didnt understood much. Maybe will give it another try since im studying pascal in school and understand some basic things now.UF ComitteD wrote:cool, I am happy to see that this clan is doing stuff like that. Thats the reason I joined i4Ni before this to be with my designer friends. I wished I would have joined UF instead of i4Ni before. At least I am here now:)
UF Beazt- UF Member
- Posts : 968
Join date : 2009-09-11
Age : 29
Location : Helsinki
Re: C# - Lesson 5b - Visual C# overview + our first real programme
Well if anyone needs somekind help with programming or just wants to talk about that, then you can contact me, since im on programming atm. You can ask about HTML, CSS, Javascript, C++, cause i have learned those. Im not saying that im expert on those, but somehwere in middle.

UF Vishna- UF Member
- Posts : 560
Join date : 2010-04-30
Age : 30
Location : Estonia
Re: C# - Lesson 5b - Visual C# overview + our first real programme
did you learned them by yourself or had a course or something?
UF Beazt- UF Member
- Posts : 968
Join date : 2009-09-11
Age : 29
Location : Helsinki
Re: C# - Lesson 5b - Visual C# overview + our first real programme
Mostly myself, but C++ learned in school in Mechatronics and Robotics class
UF Vishna- UF Member
- Posts : 560
Join date : 2010-04-30
Age : 30
Location : Estonia
Re: C# - Lesson 5b - Visual C# overview + our first real programme
UF Beazt wrote:Not sure is anyone doing really this stuff. Xorax wrote this tutorial several months ago. I tried to learn at start but didnt understood much. Maybe will give it another try since im studying pascal in school and understand some basic things now.UF ComitteD wrote:cool, I am happy to see that this clan is doing stuff like that. Thats the reason I joined i4Ni before this to be with my designer friends. I wished I would have joined UF instead of i4Ni before. At least I am here now:)
Just to say, it is way more harder to study this, when you dont have an actual person right there to explain the differances to you, because it can be quite confusing at times. If you are considering of doing something, then Vishna will be probably a lot of help to you. That is if you are consider doing something in C++ .
UF Spartan19423- UF Admin
- Posts : 1635
Join date : 2009-01-28
Age : 30
Location : Estonia
Re: C# - Lesson 5b - Visual C# overview + our first real programme
I would imagine different programming languages are similar, I mean they have different commands and functions but logical thinking and basics are still pretty much the same.
But im not really considering learning c++ now. Im busy learning proper modeling in max so its enough of new information for me. especially in summer
But im not really considering learning c++ now. Im busy learning proper modeling in max so its enough of new information for me. especially in summer

UF Beazt- UF Member
- Posts : 968
Join date : 2009-09-11
Age : 29
Location : Helsinki
Learn More on C#
I have been learning from this "Book" for a while i found it "Somewhere" and i think you guys will like it
Download Its a .Pdf
i know the post is old but if you see it you will like it i think
Download Its a .Pdf
i know the post is old but if you see it you will like it i think
Shawnm1700- Guest
- Posts : 2
Join date : 2011-06-08
Age : 29
Location : In your moms bed ^_^
Re: C# - Lesson 5b - Visual C# overview + our first real programme
After a long time of trying, I realized that programming and stuff maybe me not my thing.
It is just, quite complicated
Maybe I might try to use the book
It is just, quite complicated

Maybe I might try to use the book

UF Garanas- UF Member
- Posts : 998
Join date : 2011-01-10
Age : 29
Location : Netherlands
Re: C# - Lesson 5b - Visual C# overview + our first real programme
Hmm seems thorough, maybe I will try it to someday, when I go back to C langeuage. Atm I'm learning Java.
UF Vishna- UF Member
- Posts : 560
Join date : 2010-04-30
Age : 30
Location : Estonia
Re: C# - Lesson 5b - Visual C# overview + our first real programme
Java seems to be the future
, But it is quite hard if you're asking me.
I guess languages aren't my thing

I guess languages aren't my thing

UF Garanas- UF Member
- Posts : 998
Join date : 2011-01-10
Age : 29
Location : Netherlands
Re: C# - Lesson 5b - Visual C# overview + our first real programme
Yeah, English is one if themUF Garanas wrote:Java seems to be the future, But it is quite hard if you're asking me.
I guess languages aren't my thing

UF WiredUp4Fun- UF Admin
- Posts : 1265
Join date : 2009-08-18
Age : 34
Location : Australia
Re: C# - Lesson 5b - Visual C# overview + our first real programme
UF Garanas wrote:Java seems to be the future, But it is quite hard if you're asking me.
I guess languages aren't my thing
Yeah its hardest what I have learned, but if you have learned atleast C/C#/C++ then its easier to understand. But I agree, that you need teacher. Atleast for couple of first lesson, to get the logic behind java. Hardest thing is IMO debugging. It can give so complex errors what I can only solve with teachers help.
Maybe, maybe I can put up some tutorials in future, but not atm, need to learn more.
UF Vishna- UF Member
- Posts : 560
Join date : 2010-04-30
Age : 30
Location : Estonia
Re: C# - Lesson 5b - Visual C# overview + our first real programme
UF WiredUp4Fun wrote:Yeah, English is one if themUF Garanas wrote:Java seems to be the future, But it is quite hard if you're asking me.
I guess languages aren't my thinghaha
When you're asking me.
That should do it. :p
Debugging isn't the hardest thing, the hardest thing is preventing bugs before you make them!
And a teacher would be lovely, but these things are (mostly, as far I can see) only given at universities / the other type of high school.
UF Garanas- UF Member
- Posts : 998
Join date : 2011-01-10
Age : 29
Location : Netherlands
Re: C# - Lesson 5b - Visual C# overview + our first real programme
If you want to be so specific, then yes. And other type high school? Well it just depends I guess. I'm learning in normal school, wich dont have only one specification. There are selection curses and well, our school has decided to make different kind of selection courses every year. So yeah maybe I'm lucky. Previous year robotics and this year programming. 

UF Vishna- UF Member
- Posts : 560
Join date : 2010-04-30
Age : 30
Location : Estonia

» C# - Lesson 5a - Visual C# overview + our first real programme
» C# - Lesson 3 - Conditions
» C# - Lesson 4 - Iterations
» C# - Lesson 1 - General Language Look
» C# - Lesson 2 - Variables & Strings
» C# - Lesson 3 - Conditions
» C# - Lesson 4 - Iterations
» C# - Lesson 1 - General Language Look
» C# - Lesson 2 - Variables & Strings
Page 1 of 1
Permissions in this forum:
You cannot reply to topics in this forum
|
|